ProxySession Class Reference
<class description="" goes="" here=""> <short description=""> More...
#include <ProxySession.hpp>
Inheritance diagram for ProxySession:
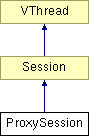
Public Member Functions | |
ProxySession (int socket, const struct sockaddr_in *client, CacheManager *cm, AdmissionControl *adm, bool allowRTX, SessionCounter *counter=NULL, TerminalCapabilities *defTC=NULL) | |
socket: the raw socket for initializing the session control channel. | |
bool | getOptions (const Url *fileName, const char *remaining) |
bool | setOptions (const Url *fileName, const char *remaining) |
bool | options (const Url *fileName, const char *remaining) |
bool | connect (const Url *fileName, const char *remaining) |
bool | setup (const Url *fileName, const char *remaining) |
bool | play (const Url *fileName, const char *remaining) |
bool | pause (const Url *fileName, const char *remaining) |
bool | tearDown (int sessionKey, bool immediate=false, const Url *fileName=NULL, const char *remaining=NULL) |
teardowns the session specified with sessionKey (if no fileName and no reminaing is specified. | |
void | run () |
void | setUrl (const Url *uri, bool makeExactMatch=true) |
deep-copies uri, sets mp4Stream by asking the cacheManager | |
SessionState | getState () const |
Protected Member Functions | |
int | readData (char *buffer, int numBytes) |
sets output at the client | |
Protected Attributes | |
ServerSession * | serverSession |
std::map< u32, u32, struct intCompare > | sessionTranslator |
CacheManager * | cm |
char | buffer [MSG_BUFFER_SIZE+1] |
char | bufferBackup [MSG_BUFFER_SIZE+1] |
sockaddr_in * | server |
bool | rtxEnabledToServer |
bool | rtxEnabledToClient |
bool | rtxEnabled |
does this proxysession support retransmissions | |
bool | delMp4Stream |
should the created mp4Stream object be deleted | |
char * | playerId |
list< rtx_info * > | rtxInfoServer |
bool | insertMp4StreamIntoCM |
is true, when the Mp4Stream Info object is newly created or updated and has to be inserted into the CacheManager | |
bool | dualMiss |
both, the original input stream and the adapted output stream, have to be inserted into the CacheManager | |
bool | dontCacheDualMiss |
bool | noDescribeSeenYet |
SessionCounter * | counter |
Detailed Description
<class description="" goes="" here=""> <short description="">
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- ProxySession.hpp,v 1.26 2006/01/20 15:37:54 mkropfbe Exp
Definition at line 135 of file ProxySession.hpp.
Constructor & Destructor Documentation
|
socket: the raw socket for initializing the session control channel. the client info is deep-copied
References delMp4Stream, dualMiss, and insertMp4StreamIntoCM.
|
Member Function Documentation
|
deep-copies uri, sets mp4Stream by asking the cacheManager
Implements Session. Definition at line 1289 of file ProxySession.cpp. References ContainerInfo::clone(), ReferenceCounter::decrease(), delMp4Stream, CacheManager::findMetaObject(), MetaObject::findMp4Stream(), TerminalCapabilities::getColorDisplay(), TerminalCapabilities::getDisplayHeight(), TerminalCapabilities::getDisplayRefreshRate(), TerminalCapabilities::getDisplayWidth(), ContainerInfo::getFileSize(), ContainerInfo::getFirstVisualES(), TerminalCapabilities::getMaxDecoderBitRateInBit(), Adaptor::getName(), TerminalCapabilities::getNetworkCapacityInByte(), ContainerInfo::getUsageCounter(), CacheManager::getVideo(), insertMp4StreamIntoCM, CacheManager::lockCache(), CacheManager::reserveDiskSpace(), Adaptor::setESInfo(), Url::toString(), and CacheManager::unlockCache().
|
|
teardowns the session specified with sessionKey (if no fileName and no reminaing is specified. Otherwise remaining will be parsed for the sessionkey, and the first param is ignored Implements Session. Definition at line 1124 of file ProxySession.cpp. References ReferenceCounter::decrease(), RTSP::generateOk(), RTSP::generateSessionNotFound(), RTSP::getBuffer(), ESInfo::getFrameStatistic(), ESInfo::getInput(), DataSink::getOutput(), BitField::getPercentageSetBits(), ESInfo::getUsageCounter(), RTSP::incSeqNr(), and ESInfo::setCompleteState().
|
The documentation for this class was generated from the following files: