Rtp Class Reference
<class description="" goes="" here=""> <short description=""> More...
#include <Rtp.hpp>
Inheritance diagram for Rtp:
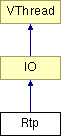
Public Member Functions | |
Rtp (const char *url, int remotePrt, const char *address, int localPrt, ESInfo *es, PacketizationLayer *packetization, bool writeOnly, const TerminalCapabilities *tc, GlobalTimer *globalTimer, Statistics *statistics, bool allowVopTimeDetection=true) | |
creates an RTP object | |
Statistics * | getStatistics () |
void | setESInfo (ESInfo *new_es) |
ESInfo * | getESInfo () |
Frame * | getFrame () |
returns a frame if one complete frame is available, otherwise null is returned. | |
int | writeFrame (Frame *frm, ESInfo *out_es=NULL) |
just adds a frame to the send buffer. | |
void | resendHeader () |
if this is called, the next sent frame will also include a decoder header this could be used for multicast sessions | |
void | run () |
this thread works on the read buffer, using the estimated avail. | |
bool | open () |
opens the IO connection. | |
State | play (double prefetchTime) |
State | pause () |
State | mute () |
bool | close (bool immediate=false) |
closes the IO class. | |
int | getLocalPort () const |
int | getBufferFillLevelInPercent () const |
returns preQ buffer fill in percent | |
int | getBufferFillLevel () const |
returns a value from 0..100 indicating buffer usage | |
int | getBufferFillLevelInBytes () const |
returns preQ buffer fill in Bytes | |
int | flushBuffer (long from_ts=0, long to_ts=-1) |
flushes the preQ buffer from starting TimeStamp to end TS | |
bool | isInput () |
bool | isOutput () |
bool | destroy () |
you can't delete a remote url :-) will always return true | |
const char * | getURL () const |
returns a pointer to the local file name or an URL | |
char * | getSSRC () |
returns NULL if the session was not opened, Otherwise, a char* containing a 08x hexValue | |
void | setRtxInfo (const rtx_info *rtx) |
sets a shallow copy of | |
const rtx_info * | getRtxInfo () const |
bool | setToFrameNumber (u32 frameNumber) |
repositions the IO class to the given frame. | |
bool | setEndFrameNumber (u32 frameNumber) |
sets the last frame that should be sent. | |
Protected Types | |
typedef Rtp::NetPacket_t | NetPacket_t |
enum | QOrder { Q_HEAD, Q_TAIL, Q_TS, Q_SEQ } |
Protected Member Functions | |
bool | insertToPreQ (u8 *payload, long pl_size, Frame::FrameType type, long vop_size, int prio, u32 rtpTimestamp, u32 fragCounter, bool lastFrag, u32 rtp_seq, QOrder qo) |
inserts an SL packet to the preQ in the given order | |
bool | reinsertToPreQ (NetPacket_t *p, uint rtp_seq) |
reinserts a SL packet for retransmission to the head of the preQ | |
bool | addToPreQ (NetPacket_t *p, QOrder qo, bool pushback=false) |
adds a NetPacket to the head or tail of the preQ (this is used from insertToPreQ or for retransmissions) or sorted by a frames' timestamp (used for receiving RTP) | |
NetPacket_t * | popPreQ (bool blocking=true) |
block or get the first packet off the preQ this does neither free the packet, nor the packet payload! | |
void | calcAdaptPreQValues (int net_bw) |
it decides, how many stream seconds should be brought thru in this second (this is needed to fill up the buffers or send a better quality even if the network does not allow it --> only if client buffers are full!) it also sets the percentage of adaptation | |
void | markFullFramePartially (bool dropped, bool sent, u32 ts) |
searches all packets with the same TS (so they belong to one single frame) and marks them all as partially_dropped or partially_sent this is used for better adaptPreQ() results (it has to adapt full frames, not packets) | |
void | adaptPreQ (int net_bw) |
marks unimportant packets for the next streamout sec, according to their priority marks packets for the next streamout second starting from the preQ head. | |
int | getNextPreQ_TS () |
bool | pushNetPacket (NetPacket_t *p) |
NetPacket_t * | popNetPacket (uint16_t rtp_seq) |
NetPacket_t * | popNetPacketByRTXseq (uint16_t rtx_rtp_seq) |
void | sendQupdate (long streamout_sec) |
scans the SendQ for already ack'd or out-dated frames and releases them. | |
void | preQupdate () |
scans the preQ for missing packets and requests their retransmission since in the client, not frame type is known, EVERY missing packet is reqeusted... | |
int | doSend (NetPacket_t *p) |
void | sendThread () |
void | readThread () |
void | ucl_update (u32 ts, u32 timeout) |
calls UCL lib for RTP,RTCP updates and callback functions (recv data) | |
Static Protected Member Functions | |
void | RtpCallback (struct rtp *session, rtp_event *e) |
void | fb_print (struct rtp *session, uint32_t ssrc, rtcp_fb *fb) |
void | fb_handle (struct rtp *session, uint32_t ssrc, rtcp_fb *fb) |
void | rtp_data_handle (struct rtp *session, uint32_t ssrc, rtp_packet *packet) |
Protected Attributes | |
Rtp::PreQ_t | preQ |
Rtp::SendQ_t | sendQ |
Statistics * | stats |
rtp * | session |
uint32_t | sender_ssrc |
int | remotePort |
int | localPort |
char * | remoteAddress |
bool | writeToNetwork |
bool | firstFrame |
struct { | |
int numProcessedFrames | |
int seqno | |
int act_ts | |
} | IOFrameStat |
GlobalTimer * | globalTimer |
PacketizationLayer * | pLayer |
ESInfo * | es |
char * | url |
rtx_info * | rtx |
bool | feedbackEnabled |
bool | rtxEnabled |
int64_t | last_rr_seq_no |
int64_t | last_rr_sum_pkts_lost |
double | last_rr_lost_fract |
double | last_rr_received |
int64_t | first_rtp_seq |
uint16_t | last_rtp_seq |
int | ts_offset |
int64_t | first_rtx_rtp_seq |
uint16_t | last_rtx_rtp_seq |
int | interarrival_time |
int | net_bw_before_superdrain |
int | real_bw |
bool | threadWasPaused |
bool | allowVopTimeDetection |
Static Protected Attributes | |
const int | TTL = 16 |
const int | MAX_PAYLOAD_SIZE = 65000 |
const int | RTP_PAYLOAD_TYPE = 96 |
Detailed Description
<class description="" goes="" here=""> <short description="">
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- Rtp.hpp,v 1.30 2006/01/26 11:42:00 mkropfbe Exp
Definition at line 108 of file Rtp.hpp.
Constructor & Destructor Documentation
|
creates an RTP object
References Statistics::getBaseStreamBW(), TerminalCapabilities::getNetworkCapacityInByte(), Statistics::getStreamBW(), Statistics::setBaseStreamBW(), and IO::setState().
|
Member Function Documentation
|
marks unimportant packets for the next streamout sec, according to their priority marks packets for the next streamout second starting from the preQ head. if new data or RTX makes the preQ dirty, a new marking is done, now starting at exactly from the new preQ head. RTX is never marked for adaptation. it has to adapt full frames, not packets. to do so, it looks at partially_sent and partially_dropped markers of packets
References Statistics::getAdaptRate(), Statistics::getAdaptSecs(), Statistics::getBufAheadSec(), and Statistics::getStreamBW().
|
|
it decides, how many stream seconds should be brought thru in this second (this is needed to fill up the buffers or send a better quality even if the network does not allow it --> only if client buffers are full!) it also sets the percentage of adaptation
References Statistics::getAdaptRate(), Statistics::getAdaptSecs(), Statistics::getBufAheadSec(), Statistics::getStreamBW(), Statistics::getStreamoutSec(), Statistics::setAdaptRate(), and Statistics::setAdaptSecs().
|
|
closes the IO class.
Implements IO. Definition at line 673 of file Rtp.cpp. References PortGenerator::closePortPair(), popPreQ(), sendQupdate(), and IO::setState(). Referenced by getFrame().
|
|
returns a frame if one complete frame is available, otherwise null is returned. This function is typically blocking. Don't use a NULL return value to conclude STREAMEOF, always check with getState()! Implements IO. Definition at line 770 of file Rtp.cpp. References addToPreQ(), close(), flushBuffer(), Frame::getAU(), Statistics::getBufAheadSec(), ESInfo::getDuration(), Statistics::getFirstPacketTime(), Statistics::getFirstPacketTS(), Statistics::getHighestPacketTS(), ESInfo::getMediaTimeScale(), ESInfo::getNumberOfMediaSamples(), Statistics::getPrefetchedSecs(), Statistics::getStillToPrefetchSecs(), Frame::getType(), ESInfo::isAudioStream(), ESInfo::isVisualStream(), popPreQ(), Frame::setAU(), Statistics::setBufAheadSec(), ESInfo::setDecoderConfig(), Frame::setMediaTimeScale(), Statistics::setPrefetchedSecs(), GlobalTimer::setPrefetching(), IO::setState(), and Statistics::setStillToPrefetchSecs().
|
|
|
|
opens the IO connection. State is set to OPENING. Depending on the underlying QIODevice, a network connection or a file connection is established. When the connection is ready for use, State is OPEN and the run thread is started. Implements IO. Definition at line 268 of file Rtp.cpp. References ESInfo::getAvgBandwidth(), Statistics::getClientPreQMaxSize(), PortGenerator::getSockFromPort(), Statistics::setClientPreQMaxSize(), and IO::setState().
|
|
block or get the first packet off the preQ this does neither free the packet, nor the packet payload!
References ESInfo::getMediaTimeScale(), and ESInfo::getVOPTimeIncrement(). Referenced by close(), and getFrame().
|
|
scans the preQ for missing packets and requests their retransmission since in the client, not frame type is known, EVERY missing packet is reqeusted... Definition at line 1671 of file Rtp.cpp. References Statistics::getHighestPacketTS().
|
|
this thread works on the read buffer, using the estimated avail. bandwidth for send out rate Reimplemented from IO. Definition at line 2096 of file Rtp.cpp. References VThread::setRoundRobinScheduling().
|
|
scans the SendQ for already ack'd or out-dated frames and releases them.
References ESInfo::getMediaTimeScale(), and Statistics::getPrefetchedSecs(). Referenced by close().
|
|
sets the last frame that should be sent. Allows to specify in combination with setToFrameNumber a range of frames that should be sent. Set
Reimplemented from IO. Definition at line 448 of file Rtp.cpp. References flushBuffer(), and ESInfo::getVOPTimeIncrement().
|
|
sets a shallow copy of
Reimplemented from IO. Definition at line 2853 of file Rtp.cpp.
|
|
repositions the IO class to the given frame. Will return false in the following cases:
Reimplemented from IO. Definition at line 461 of file Rtp.cpp. References flushBuffer().
|
|
calls UCL lib for RTP,RTCP updates and callback functions (recv data)
|
|
just adds a frame to the send buffer. sending per se is handled in the run thread. If the send buffer is full, writeFrame will block.
Implements IO. Definition at line 552 of file Rtp.cpp. References PacketizationLayer::createSLPacketList(), Frame::getAU(), ESInfo::getHeaders(), Frame::getType(), ESInfo::getVOPTimeIncrement(), and insertToPreQ().
|
The documentation for this class was generated from the following files: