Session Class Reference
<class description="" goes="" here=""> <short description=""> More...
#include <Session.hpp>
Inheritance diagram for Session:
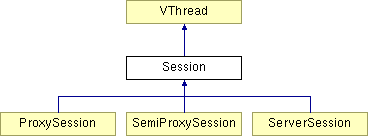
Public Types | |
enum | SessionState { SESSION_ERR, SESSION_NEW, SESSION_INITIALIZED, SESSION_ACTIVE, SESSION_PAUSED, SESSION_CLOSED } |
Public Member Functions | |
virtual bool | options (const Url *fileName, const char *remaining)=0 |
virtual bool | connect (const Url *fileName, const char *remaining)=0 |
virtual bool | setup (const Url *fileName, const char *remaining)=0 |
virtual bool | play (const Url *fileName, const char *remaining)=0 |
virtual bool | pause (const Url *fileName, const char *remaining)=0 |
virtual bool | getOptions (const Url *fileName, const char *remaining)=0 |
virtual bool | setOptions (const Url *fileName, const char *remaining)=0 |
virtual bool | tearDown (int sessionKey, bool immediate=false, const Url *fileName=NULL, const char *remaining=NULL)=0 |
teardowns the session specified with sessionKey (if no fileName and no reminaing is specified. | |
virtual void | setUrl (const Url *uri, bool makeExactMatch=true)=0 |
deep-copies uri, sets mp4Stream by asking the cacheManager | |
const Url * | getUrl () const |
bool | setRtxInfoForES (u32 esId, rtx_info *rtx) |
creates a rtx_group for the given input params | |
rtx_group * | getRtxGroup (u32 esId) |
returns an rtx_group for the esId, or NULL | |
std::list< rtx_group * > * | getAllRtxGroups () |
const struct sockaddr_in * | getDataSinkInfo () const |
void | setDataChannel (DataChannel *dc) |
simply appends | |
Adaptor * | getSuggestedAdaptor () |
const std::list< DataChannel * > & | getDataChannels () const |
DataChannel * | getDataChannel (int esId) |
searches an existing DataChannel within this session for the given | |
ContainerInfo * | getContainerInfo () const |
void | setTerminalCapabilities (TerminalCapabilities *tc) |
const TerminalCapabilities * | getTerminalCapabilities () const |
int | getControlSocket () const |
bool | sendResponse (const char *str, int bytes) |
writes the string to the session control channel | |
int | readRequest (char *str, const int MAX_BYTES) |
reads at most | |
RTSP * | getProtocol () |
KnownProtocols | determineProtocol (const char *request) |
returns the known protocol or PROTO_UNKNOWN | |
Protected Member Functions | |
DataChannel * | find (int esId) |
searches for an ES its DataChannel. | |
Protected Attributes | |
GlobalTimer * | globalTimer |
ReferenceCounter * | gtRefCount |
SharedAdaptor * | gtSharedAdapt |
ResourceUsage | resUsage |
int | sessionControlChannel |
SessionState | state |
TerminalCapabilities * | termCap |
UserPreferences * | userPref |
ContainerInfo * | mp4Stream |
list< rtx_group * > | rtxInfo |
stores for each ES an optional retransmission wish | |
list< DataChannel * > | channels |
the List of all DataChannels that this Session manages. | |
Adaptor * | suggestedAdaptor |
sockaddr_in * | client |
u32 | clientId |
RTSP * | prot |
Url * | url |
the url of the source/destination file | |
char * | serverID |
bool | canAdmitRequest |
Detailed Description
<class description="" goes="" here=""> <short description="">
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- Session.hpp,v 1.16 2006/01/20 15:37:54 mkropfbe Exp
Definition at line 79 of file Session.hpp.
Member Function Documentation
|
searches for an ES its DataChannel. returns NULL on failure |
|
searches an existing DataChannel within this session for the given
References channels.
|
|
reads at most
|
|
simply appends
References channels.
|
|
deep-copies uri, sets mp4Stream by asking the cacheManager
Implemented in ProxySession, ServerSession, and SemiProxySession.
|
|
teardowns the session specified with sessionKey (if no fileName and no reminaing is specified. Otherwise remaining will be parsed for the sessionkey, and the first param is ignored Implemented in ProxySession, ServerSession, and SemiProxySession.
|
Member Data Documentation
|
the List of all DataChannels that this Session manages. 1 DataChannel = 1 ESDefinition at line 162 of file Session.hpp. Referenced by getDataChannel(), and setDataChannel(). |
The documentation for this class was generated from the following files: