Adaptor Class Reference
<class description="" goes="" here=""> <short description=""> More...
#include <Adaptor.hpp>
Inheritance diagram for Adaptor:
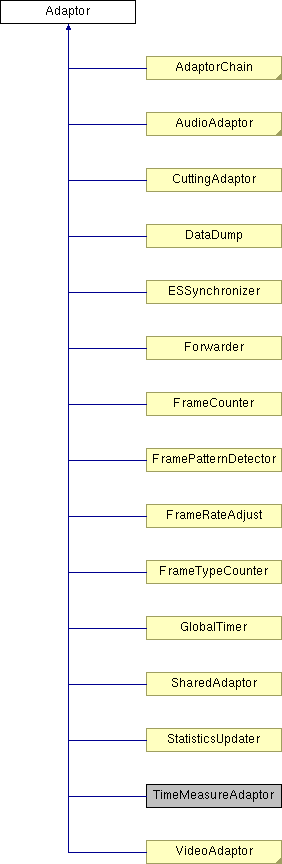
Public Member Functions | |
virtual list< Frame * > | adapt (Frame *frm) |
Accepts as input a frame, and checks if it can adapt the frame, If the frame is modified, a new Frame object is created and inserted into the return list. | |
virtual list< Frame * > | close () |
Close and destroy an Adaptor. | |
virtual Adaptor * | clone ()=0 |
Create a shallow copy of the Adaptor. | |
virtual void | initialize ()=0 |
Initialize internal data structures. | |
virtual const char * | getName () |
Return the name of the Adaptor. | |
virtual void | setESInfo (ESInfo *esi) |
Sets a reference to an ESInfo object. | |
virtual ESInfo * | getESInfo () |
virtual u32 | getTranscodingCosts () const =0 |
returns adaptation costs (CPU only) per second! For visual streams they are expressed in pixels/seconds. | |
Protected Attributes | |
bool | initialized |
char | name [128] |
the name of the Adaptor, Workaround for broken instanceof in compilers and used for pretty-printing. | |
ESInfo * | es |
Detailed Description
<class description="" goes="" here=""> <short description="">
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- Adaptor.hpp,v 1.14 2006/01/20 15:37:17 mkropfbe Exp
Definition at line 69 of file Adaptor.hpp.
Member Function Documentation
|
Accepts as input a frame, and checks if it can adapt the frame, If the frame is modified, a new Frame object is created and inserted into the return list. This new frame is returned only, if the size of the payload is larger than zero. If the frame was not modified, it is also inserted into the list. For more complex adaptors, it will happen, that the adaptor caches up one complete GOP and returns the full GOP in network order. A caching adaptor has to create deep-copies for each frames. Never directly modify the payload of the input frm, always create copies!!!
Reimplemented in AdaptorChain, CuttingAdaptor, DataDump, ESSynchronizer, Forwarder, FrameCounter, FramePatternDetector, FrameRateAdjust, FrameRotate, FrameTypeCounter, GlobalTimer, MadMP3Decoder, MP3Encoder, MP4audioDecoder, MP4Decoder, MP4Encoder, PSNR, SharedAdaptor, StatisticsUpdater, StrongTemporalAdaptor, TemporalAdaptor, TheoraEncoder, Visualizer, VorbisEncoder, YUVColorReductionAdaptor, YUVinYUVoverlay, YUVScalingAdaptor, and YUVSpatialReductionAdaptor. Definition at line 88 of file Adaptor.hpp. Referenced by SharedAdaptor::adapt(), AdaptorChain::adapt(), DataChannel::run(), and DataSink::send().
|
|
Create a shallow copy of the Adaptor. Creates an Adaptor with the same setup (without copying the current status).
Implemented in ColorReductionAdaptor, CuttingAdaptor, DataDump, ESSynchronizer, Forwarder, FrameCounter, FramePatternDetector, FrameRateAdjust, FrameRotate, FrameTypeCounter, GlobalTimer, MadMP3Decoder, MP3Encoder, MP4audioDecoder, MP4Decoder, MP4Encoder, PSNR, QualityReductionAdaptor, SharedAdaptor, SpatialReductionAdaptor, StatisticsUpdater, StrongTemporalAdaptor, TemporalAdaptor, TheoraEncoder, Visualizer, VorbisEncoder, YUVColorReductionAdaptor, YUVinYUVoverlay, YUVScalingAdaptor, YUVSpatialReductionAdaptor, AudioAdaptorChain, and VideoAdaptorChain.
|
|
Close and destroy an Adaptor. Releases all internally buffered frame objects, and deallocate all allocated memory. The Adaptor must not be used after calling the close method!!!
Reimplemented in AdaptorChain, CuttingAdaptor, DataDump, ESSynchronizer, FrameCounter, FramePatternDetector, FrameRateAdjust, FrameTypeCounter, GlobalTimer, MadMP3Decoder, MP3Encoder, MP4audioDecoder, MP4Decoder, MP4Encoder, PSNR, StatisticsUpdater, StrongTemporalAdaptor, TemporalAdaptor, Visualizer, VorbisEncoder, YUVColorReductionAdaptor, YUVinYUVoverlay, YUVScalingAdaptor, and YUVSpatialReductionAdaptor. Definition at line 105 of file Adaptor.hpp. Referenced by DataSink::close(), and DataChannel::run().
|
|
Return the name of the Adaptor. Do use the same name as the classname (workaround for broken isInstanceOf in most compilers).
References name. Referenced by DataChannel::run(), DataSink::send(), and ProxySession::setUrl().
|
|
returns adaptation costs (CPU only) per second! For visual streams they are expressed in pixels/seconds. Call this only on INITIALIZED adaptors!!!! Otherwise costs will be way too high. Implemented in AdaptorChain, CuttingAdaptor, DataDump, ESSynchronizer, Forwarder, FrameCounter, FramePatternDetector, FrameRateAdjust, FrameRotate, FrameTypeCounter, GlobalTimer, MadMP3Decoder, MP3Encoder, MP4audioDecoder, MP4Decoder, MP4Encoder, PSNR, SharedAdaptor, StatisticsUpdater, StrongTemporalAdaptor, TemporalAdaptor, TheoraEncoder, Visualizer, VorbisEncoder, YUVColorReductionAdaptor, YUVinYUVoverlay, YUVScalingAdaptor, and YUVSpatialReductionAdaptor.
|
|
Initialize internal data structures.
Implemented in AdaptorChain, ColorReductionAdaptor, CuttingAdaptor, DataDump, ESSynchronizer, Forwarder, FrameCounter, FramePatternDetector, FrameRateAdjust, FrameRotate, FrameTypeCounter, GlobalTimer, MadMP3Decoder, MP3Encoder, MP4audioDecoder, MP4Decoder, MP4Encoder, PSNR, QualityReductionAdaptor, SharedAdaptor, SpatialReductionAdaptor, StatisticsUpdater, StrongTemporalAdaptor, TemporalAdaptor, TheoraEncoder, Visualizer, VorbisEncoder, YUVColorReductionAdaptor, YUVinYUVoverlay, YUVScalingAdaptor, and YUVSpatialReductionAdaptor.
|
|
Sets a reference to an ESInfo object. Adaptors are allowed to change ESInfo objects. If you don't want that, pass an es->clone(). NEVER delete this es in the destructor of your Adaptor! Reimplemented in CuttingAdaptor, DataDump, ESSynchronizer, Forwarder, FrameCounter, FramePatternDetector, FrameRateAdjust, FrameRotate, FrameTypeCounter, GlobalTimer, SharedAdaptor, StatisticsUpdater, YUVinYUVoverlay, AudioAdaptorChain, and VideoAdaptorChain. Definition at line 138 of file Adaptor.hpp. Referenced by ContainerDemux::demultiplexAndUpdateIO(), ProxySession::setUrl(), and DataChannel::visualizeCaching().
|
Member Data Documentation
|
the name of the Adaptor, Workaround for broken instanceof in compilers and used for pretty-printing. Do not forget to initialize this value in your subclassedDefinition at line 155 of file Adaptor.hpp. Referenced by getName(). |
The documentation for this class was generated from the following file: