MP4Encoder Class Reference
Implementation of Adaptor that takes UncompressedVideoFrame as input and returns an CompressedVideoFrame as output. <short description="">. More...
#include <MP4Encoder.hpp>
Inheritance diagram for MP4Encoder:
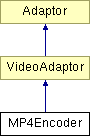
Public Member Functions | |
MP4Encoder (VideoESInfo *pESInfo, u32 targetBitRate, u32 frameRate, u32 uiKeyFrameInterval=300, u32 numBFrames=0, UncompressedVideoFrame::ColorSpace colorSpace=UncompressedVideoFrame::ColorSpaceI420, MP4Decoder::eCoderType coderType=MP4Decoder::XVID, enum CodecID outputFormat=CODEC_ID_MPEG4, char *mp4EncoderConfig=NULL) | |
Constructor. | |
virtual | ~MP4Encoder () |
Destructor. | |
list< Frame * > | adapt (Frame *frm) |
YUVFrame in - MPGFrame out. | |
list< Frame * > | close () |
Flush all buffered MPGFrame objects (if any). | |
Adaptor * | clone () |
Not implemented. | |
void | initialize () |
Initialize internal data structures. | |
void | setCoderType (MP4Decoder::eCoderType ct) |
u32 | getTranscodingCosts () const |
returns adaptation costs (CPU only). | |
Static Public Member Functions | |
ffmpegColorSpace | mapToFFMPEGColorspace (UncompressedVideoFrame::ColorSpace colorSpace) |
Mapping function between MP4Decoders colorspace (defined in meColorSpace) and FFMPEG codec colorspace. | |
u8 * | createNewHeader (VideoESInfo *vid, u32 targetBitRate, u32 width, u32 height, u32 uiKeyFrameInterval, int framerate, u32 *headerSize) |
Detailed Description
Implementation of Adaptor that takes UncompressedVideoFrame as input and returns an CompressedVideoFrame as output. <short description="">.
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- MP4Encoder.hpp,v 1.16 2005/05/31 06:24:58 mkropfbe Exp
Definition at line 67 of file MP4Encoder.hpp.
Constructor & Destructor Documentation
|
Constructor. Creates a MP4Encoder instance. Do set attributes of pESInfo object carefully. Width, Height, and FPS must match the YUV input!
Referenced by clone(). |
Member Function Documentation
|
YUVFrame in - MPGFrame out. Input YUVFrame object will be deleted in this method! Adaptor implementation for YUVFrame objects.
Reimplemented from Adaptor. Definition at line 106 of file MP4Encoder.cpp. References CompressedVideoFrame::detectFrameType(), Frame::getAU(), Frame::getMediaTimeScale(), Frame::getType(), initialize(), Frame::setAU(), and Frame::setMediaTimeScale().
|
|
Not implemented.
Implements Adaptor. Definition at line 222 of file MP4Encoder.cpp. References MP4Encoder().
|
|
Flush all buffered MPGFrame objects (if any).
Reimplemented from Adaptor. Definition at line 214 of file MP4Encoder.cpp.
|
|
returns adaptation costs (CPU only). Decoding costs depend directly on the number of out YUV pixels and frames decoded per second (FIXME: codec not supported) Implements Adaptor. Definition at line 381 of file MP4Encoder.cpp.
|
|
Initialize internal data structures.
Implements Adaptor. Definition at line 80 of file MP4Encoder.cpp. Referenced by adapt().
|
|
Mapping function between MP4Decoders colorspace (defined in meColorSpace) and FFMPEG codec colorspace.
References UncompressedVideoFrame::ColorSpace.
|
The documentation for this class was generated from the following files: