PSNR Class Reference
Adaptor class PSNR calculates psnr for each frame and provides overall statistics <short description="">. More...
#include <PSNR.hpp>
Inheritance diagram for PSNR:
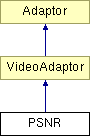
Public Member Functions | |
PSNR (VideoESInfo *esi, YUVStreamIO *OrigYUVStream, Statistics *statistics, FILE *psnrFP=NULL) | |
list< Frame * > | adapt (Frame *frm) |
Accepts as input a frame, and checks if it can adapt the frame, If the frame is modified, a new Frame object is created and inserted into the return list. | |
Adaptor * | clone () |
Create a shallow copy of the Adaptor. | |
void | initialize () |
Initialize internal data structures. | |
list< Frame * > | close () |
Close and destroy an Adaptor. | |
u32 | getTranscodingCosts () const |
returns adaptation costs (CPU only) | |
Protected Attributes | |
YUVStreamIO * | yuvOrig |
AU | lastAU |
u8 * | lastPayload |
UncompressedVideoFrame * | dummyFrame |
Statistics * | stats |
double | sumPSNR |
int | numFrames |
int | numFramesNotInf |
FILE * | psnrInfo |
Detailed Description
Adaptor class PSNR calculates psnr for each frame and provides overall statistics <short description="">.
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- PSNR.hpp,v 1.11 2006/01/20 15:37:17 mkropfbe Exp
Definition at line 64 of file PSNR.hpp.
Member Function Documentation
|
Accepts as input a frame, and checks if it can adapt the frame, If the frame is modified, a new Frame object is created and inserted into the return list. This new frame is returned only, if the size of the payload is larger than zero. If the frame was not modified, it is also inserted into the list. For more complex adaptors, it will happen, that the adaptor caches up one complete GOP and returns the full GOP in network order. A caching adaptor has to create deep-copies for each frames. Never directly modify the payload of the input frm, always create copies!!!
Reimplemented from Adaptor. Definition at line 90 of file PSNR.cpp. References UncompressedVideoFrame::computePSNR(), Frame::getAU(), YUVStreamIO::getFrame(), VideoFrame::getHeight(), Frame::getMediaTimeScale(), Statistics::getPlayoutSec(), Statistics::getPlayoutSecFPS(), Statistics::getPlayoutSecPSNR(), VideoFrame::getWidth(), Statistics::incPlayoutSecPSNR(), initialize(), Frame::setAU(), Frame::setMediaTimeScale(), and Frame::unsetAU().
|
|
Create a shallow copy of the Adaptor. Creates an Adaptor with the same setup (without copying the current status).
Implements Adaptor. Definition at line 78 of file PSNR.hpp.
|
|
Close and destroy an Adaptor. Releases all internally buffered frame objects, and deallocate all allocated memory. The Adaptor must not be used after calling the close method!!!
Reimplemented from Adaptor. Definition at line 82 of file PSNR.hpp.
|
|
Initialize internal data structures.
Implements Adaptor. Definition at line 79 of file PSNR.cpp. Referenced by adapt().
|
The documentation for this class was generated from the following files: