MP4audioDecoder Class Reference
<short description=""> More...
#include <MP4audioDecoder.hpp>
Inheritance diagram for MP4audioDecoder:
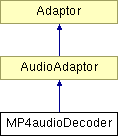
Public Types | |
typedef enum MP4audioDecoder::eCoderType | meCoderType |
Codec selection enumeration, currently available is FFMPEG only. | |
enum | MpegAudioVersionID { MPEG_VESION_2_5, RESERVED, MPEG_VERSION_2, MPEG_VERSION_1 } |
enum | MpegAudioLayerNo { RESERVed, LAYER_III, LAYER_II, LAYER_I } |
enum | ChannelMod { STEREO, JOINT_STEREO, DUAL_CHANNEL, SINGLE_CHANNEL } |
enum | Emphasis { NONE_, MS5015, RESERVEd, CCIT_J_17 } |
enum | eCoderType { XVID = 1, FFMPEG } |
Codec selection enumeration, currently available is FFMPEG only. | |
Public Member Functions | |
MP4audioDecoder (AudioESInfo *pESInfo, bool enableSwitching, meCoderType coderType=FFMPEG) | |
Creates an uninitialized MP4audioDecoder instance. | |
virtual | ~MP4audioDecoder () |
Destroys the MP4audioDecoder. | |
list< Frame * > | adapt (Frame *frm) |
Compressed frm in - uncompressed PCM frame out. | |
list< Frame * > | close () |
Flush all buffered uncompressed Frame objects (if any). | |
Adaptor * | clone () |
Not implemented. | |
void | initialize () |
Initializes the Decoder. | |
void | setCoderType (eCoderType coderType=FFMPEG) |
Codec selection. | |
u32 | getTranscodingCosts () const |
returns adaptation costs (CPU only). | |
Static Public Member Functions | |
bool | isValidAudioFrame (const u8 *payload) |
bool | isValidDecoderConfig (const u8 *payload) |
Detailed Description
<short description="">
- Author:
- Mithilesh Kumar
- Version:
- Id
- MP4audioDecoder.hpp,v 1.10 2005/04/28 08:55:47 mkropfbe Exp
Definition at line 76 of file MP4audioDecoder.hpp.
Constructor & Destructor Documentation
|
Creates an uninitialized MP4audioDecoder instance.
References meCoderType. Referenced by clone().
|
Member Function Documentation
|
Compressed frm in - uncompressed PCM frame out.
Reimplemented from Adaptor. Definition at line 212 of file MP4audioDecoder.cpp. References Frame::getAU(), initialize(), and Frame::setAU().
|
|
Not implemented.
Implements Adaptor. Definition at line 293 of file MP4audioDecoder.cpp. References MP4audioDecoder().
|
|
Flush all buffered uncompressed Frame objects (if any).
Reimplemented from Adaptor. Definition at line 279 of file MP4audioDecoder.cpp.
|
|
returns adaptation costs (CPU only). How high are audio decoding costs? assume 25% of CIF video with 25 fps (FIXME: measure it) Implements Adaptor. Definition at line 168 of file MP4audioDecoder.hpp.
|
|
Initializes the Decoder.
Implements Adaptor. Definition at line 114 of file MP4audioDecoder.cpp. Referenced by adapt().
|
|
Codec selection.
|
The documentation for this class was generated from the following files: