ESSynchronizer Class Reference
Adaptor class Synchronizer handles stream synchronization can be used for VES and AES <short description="">. More...
#include <ESSynchronizer.hpp>
Inheritance diagram for ESSynchronizer:
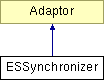
Public Member Functions | |
ESSynchronizer (ESInfo *esi, GlobalTimer *globalTimer) | |
list< Frame * > | adapt (Frame *frm) |
Blocks the calling data channel until the presentation time stamp (CTS) of the given frame matches the play-out media time returned by the associated GlobalTimer . | |
Adaptor * | clone () |
Create a shallow copy of the Adaptor. | |
void | initialize () |
Initialize internal data structures. | |
list< Frame * > | close () |
Close and destroy an Adaptor. | |
void | setPaused (bool pause) |
Blocks/deblocks the data channel this ESSynchronizer adaptor belongs to. | |
void | setESInfo (ESInfo *esi) |
Sets a reference to an ESInfo object. | |
ESInfo * | getESInfo () |
GlobalTimer * | getGlobalTimer () const |
Return associated GlobalTimer . | |
bool | setOutputRateFactor (double factor) |
Set output rate factor w.r.t. | |
double | getOutputRateFactor () const |
Returns output rate factor. | |
bool | adjust (double sec) |
Adjust the associated global timer to the given media time point. | |
virtual u32 | getTranscodingCosts () const |
returns adaptation costs (CPU only) | |
Protected Attributes | |
ESInfo * | es |
timeval | tv |
long | frmCount |
double | outputRate |
The output rate factor. |
Detailed Description
Adaptor class Synchronizer handles stream synchronization can be used for VES and AES <short description="">.
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- ESSynchronizer.hpp,v 1.15 2006/05/12 11:32:49 mkropfbe Exp
Definition at line 88 of file ESSynchronizer.hpp.
Member Function Documentation
|
Blocks the calling data channel until the presentation time stamp (CTS) of the given frame matches the play-out media time returned by the associated
If the frame lags behind the play-out time by more than Reimplemented from Adaptor. Definition at line 103 of file ESSynchronizer.cpp. References GlobalTimer::adjust(), VCondition::condWait(), GlobalTimer::getActualSec(), Frame::getAU(), ESInfo::getMediaTimeScale(), Frame::getMediaTimeScale(), VMutex::getMutexObject(), Frame::getType(), VMutex::lock(), Frame::markForDelete(), outputRate, VMutex::release(), and Frame::setMediaTimeScale().
|
|
Adjust the associated global timer to the given media time point. Note that the GlobalTimer::adjust(double) method adjusts to a play-out time point, which is different from the media time point if the output rate factor is not equal to 1.0.
References GlobalTimer::adjust(), and outputRate.
|
|
Create a shallow copy of the Adaptor. Creates an Adaptor with the same setup (without copying the current status).
Implements Adaptor. Definition at line 112 of file ESSynchronizer.hpp.
|
|
Close and destroy an Adaptor. Releases all internally buffered frame objects, and deallocate all allocated memory. The Adaptor must not be used after calling the close method!!!
Reimplemented from Adaptor. Definition at line 116 of file ESSynchronizer.hpp.
|
|
Returns output rate factor.
References outputRate.
|
|
Initialize internal data structures.
Implements Adaptor. Definition at line 70 of file ESSynchronizer.cpp.
|
|
Sets a reference to an ESInfo object. Adaptors are allowed to change ESInfo objects. If you don't want that, pass an es->clone() Reimplemented from Adaptor. Definition at line 137 of file ESSynchronizer.hpp.
|
|
Set output rate factor w.r.t. media frame rate. E.g. setting this value to 0.5 will cause a 30 Hz media stream to be forwarded with 15 Hz.
References GlobalTimer::adjust(), GlobalTimer::getActualSec(), outputRate, and GlobalTimer::setPaused().
|
|
Blocks/deblocks the data channel this ESSynchronizer adaptor belongs to.
The associated
References VMutex::lock(), VMutex::release(), and VCondition::signal(). Referenced by SDLaudioIO::open(), DSPaudioIO::open(), and DSPaudioIO::writeFrame().
|
Member Data Documentation
|
The output rate factor. 1.0 means media frame rate. Definition at line 187 of file ESSynchronizer.hpp. Referenced by adapt(), adjust(), getOutputRateFactor(), and setOutputRateFactor(). |
The documentation for this class was generated from the following files: