VideoRenderer Class Reference
This I/O class renders a gray-scale or RGB stream (via a QFrameBuffer) onto a QLabel widget. Don't forget to set the appropriate VideoFrameBuffer! More...
#include <VideoRenderer.hpp>
Inheritance diagram for VideoRenderer:
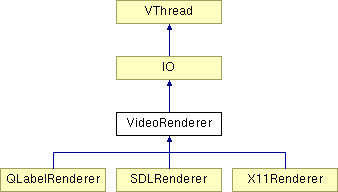
Public Member Functions | |
VideoRenderer (const VideoESInfo *es, UncompressedVideoFrame::ColorSpace colorSpace, int width, int height, bool isLabelResizable=true, bool fixAspectRatio=true, bool allowUpscaleX=false) | |
Constructor. | |
virtual | ~VideoRenderer () |
Destructor. | |
virtual Frame * | getFrame () |
Returns NULL, as this class is intended to be an output class only. | |
void | setOutputDepth (int depth) |
void | setDirectBitBlt (bool direct) |
void | setVideoFrameBuffer (VideoFrameBuffer *vfb) |
virtual int | writeFrame (Frame *frm, ESInfo *out_es=NULL) |
Renders a frame to the destination QLabel widget. | |
virtual bool | open () |
Creates internal frame buffers and checks whether the stream to be rendered matches the widget dimensions. | |
virtual bool | close (bool immediate=false) |
Does nothing but setting the object state to CLOSED. | |
virtual bool | destroy () |
Not applicable to this class. | |
virtual int | getBufferFillLevel () const |
Not applicable to this class. | |
virtual const char * | getURL () const |
Returns the fixed string VideoRenderer: . | |
virtual void | setESInfo (ESInfo *es) |
Not implemented. | |
virtual ESInfo * | getESInfo () |
Not implemented. | |
Protected Member Functions | |
bool | updateDisplayRegion () |
Update frame_info (except size ) and display_region. | |
Protected Attributes | |
char | url [MAX_STR_LEN] |
const VideoESInfo * | es |
Elementary video stream information. | |
VideoFrameBuffer * | frameBuffer |
The frame buffer. | |
UncompressedVideoFrame::ColorSpace | colorSpace |
The color space of the frames to be rendered. | |
VideoRect | frame_info |
Dimension, storage size (in bytes), and scaling factors of a decoded video frame. | |
VideoRect | out_label_info |
VideoRect | display_region |
apect-ratio adjusted region of qlabel where frames will be displayed. | |
int | outputDepth |
bool | directBitBlt |
Detailed Description
This I/O class renders a gray-scale or RGB stream (via a QFrameBuffer) onto a QLabel widget. Don't forget to set the appropriate VideoFrameBuffer!It is intended to be used as a DataSink connected to a DataChannel.
Video frames will be (down or up) scaled to fit into the QLabel widget by preserving the aspect ratio given in VideoESInfo.
The expected format of the gray-scale stream is: 1 byte per pixel.
The expected format of the RGB24 stream is: 3 bytes per pixel, in the order RGB (red, green, blue).
The expected format of the RGB32 stream is: 4 bytes per pixel, in the order BGRA (blue, green, red, alpha). This conforms to the PIX_FMT_RGBA32 format defined in ffmpeg/libavcodec/avcodec.h on little endian architectures.
- Author:
- Mario Taschwer and Michael Kropfberger
- Version:
- Id
- VideoRenderer.hpp,v 1.15 2006/01/26 11:42:00 mkropfbe Exp
Definition at line 78 of file VideoRenderer.hpp.
Constructor & Destructor Documentation
|
Constructor. If the parameters are invalid, the open() method will fail.
References UncompressedVideoFrame::ColorSpace, es, frame_info, and frameBuffer.
|
Member Function Documentation
|
Does nothing but setting the object state to CLOSED.
Implements IO. Definition at line 169 of file VideoRenderer.hpp.
|
|
Not applicable to this class.
Implements IO. Definition at line 178 of file VideoRenderer.hpp.
|
|
Not applicable to this class.
Implements IO. Definition at line 186 of file VideoRenderer.hpp.
|
|
Not implemented.
Implements IO. Definition at line 201 of file VideoRenderer.hpp.
|
|
Creates internal frame buffers and checks whether the stream to be rendered matches the widget dimensions.
Implements IO.
Reimplemented in QLabelRenderer, SDLRenderer, and X11Renderer. Definition at line 214 of file VideoRenderer.cpp. Referenced by Visualizer::adapt().
|
|
Update frame_info (except This function is not thread-safe, and is internally called by the open() method. Reimplemented in QLabelRenderer, SDLRenderer, and X11Renderer. Definition at line 111 of file VideoRenderer.cpp. References display_region, es, and frame_info. Referenced by X11Renderer::updateDisplayRegion(), SDLRenderer::updateDisplayRegion(), and QLabelRenderer::updateDisplayRegion().
|
|
Renders a frame to the destination QLabel widget.
Implements IO.
Reimplemented in QLabelRenderer, SDLRenderer, and X11Renderer. Definition at line 220 of file VideoRenderer.cpp. References colorSpace, frame_info, frameBuffer, Frame::getAU(), VideoFrameBuffer::lock(), VideoFrameBuffer::postPaintEvent(), and VideoFrameBuffer::unlock(). Referenced by Visualizer::adapt(), X11Renderer::writeFrame(), SDLRenderer::writeFrame(), and QLabelRenderer::writeFrame().
|
Member Data Documentation
|
apect-ratio adjusted region of qlabel where frames will be displayed. Frame dimensions may be smaller than display_region and out_label_info dimensions. Definition at line 247 of file VideoRenderer.hpp. Referenced by updateDisplayRegion(). |
|
Dimension, storage size (in bytes), and scaling factors of a decoded video frame.
The scaling factors are defined as
We use fixed point arithmetic with FIXED_DIGITS binary precision, so
The product Referenced by updateDisplayRegion(), VideoRenderer(), and writeFrame(). |
The documentation for this class was generated from the following files: