UncompressedVideoFrame Class Reference
<class description="" goes="" here=""> <short description=""> More...
#include <UncompressedVideoFrame.hpp>
Inheritance diagram for UncompressedVideoFrame:
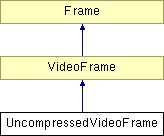
Public Types | |
typedef enum UncompressedVideoFrame::ColorSpace | ColorSpace |
Colorspace enumeration of colorspaces available. | |
enum | ColorSpace { ColorSpaceDefault = 4, ColorSpaceRGB24 = 0, ColorSpaceYV12 = 1, ColorSpaceI420 = 4, ColorSpaceRGB32 = 1000, ColorSpaceRGB565, ColorSpaceGRAY8, ColorSpaceUNKNOWN } |
Colorspace enumeration of colorspaces available. More... | |
Public Member Functions | |
UncompressedVideoFrame (FrameType t, int width, int height) | |
Constructor -----------. | |
virtual | ~UncompressedVideoFrame () |
Destructor ----------. | |
Frame::FrameType | detectFrameType () |
Determines the correctness of the buffer and extracts the frametype of the frame On any problem: FrameType ERROR_VOP is returned. | |
void | setColorSpace (ColorSpace c) |
ColorSpace | getColorSpace () |
UncompressedVideoFrame * | convertToColorSpace (ColorSpace cs) |
int | getYBuffer (u8 **buf) |
Returns the buffer with Y component of *YUVI420* of Uncompressed Video. | |
int | getUBuffer (u8 **buf) |
Returns the buffer with U component of *YUVI420* of Uncompressed Video. | |
int | getVBuffer (u8 **buf) |
Returns the buffer with V component of *YUVI420* of Uncompressed Video. | |
double | computePSNRy (UncompressedVideoFrame *f) |
computePSNRy ------------ | |
double | computePSNRu (UncompressedVideoFrame *f) |
computePSNRu ------------ | |
double | computePSNRv (UncompressedVideoFrame *f) |
computePSNRv ------------ | |
double | computePSNR (UncompressedVideoFrame *f) |
computePSNR ----------- | |
double | getPSNR () |
Static Public Member Functions | |
Frame::FrameType | detectFrameType (AU *accessUnit) |
int | mapColorSpaceToBitDepth (ColorSpace colorspace) |
Protected Member Functions | |
double | computePSNRwithOffset (UncompressedVideoFrame *f, long offset) |
Protected Attributes | |
double | psnr |
ColorSpace | colorSpace |
Detailed Description
<class description="" goes="" here=""> <short description="">
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- UncompressedVideoFrame.hpp,v 1.7 2006/06/22 13:31:17 mkropfbe Exp
Definition at line 61 of file UncompressedVideoFrame.hpp.
Member Typedef Documentation
|
Colorspace enumeration of colorspaces available. This is an excerpt of the colorspaces available in XviD (xvid.h) and/or FFmpeg (avcodec.h). so those colorSpaces are also avail in MP4Decoder
Referenced by mapColorSpaceToBitDepth(), MP4Encoder::mapToFFMPEGColorspace(), MP4Decoder::mapToFFMPEGColorspace(), MP4Decoder::mapToXVIDColorspace(), MP4Decoder::MP4Decoder(), QLabelRenderer::QLabelRenderer(), SDLRenderer::SDLRenderer(), VideoRenderer::VideoRenderer(), DataChannel::visualizeCaching(), and X11Renderer::X11Renderer(). |
Member Enumeration Documentation
|
Colorspace enumeration of colorspaces available. This is an excerpt of the colorspaces available in XviD (xvid.h) and/or FFmpeg (avcodec.h). so those colorSpaces are also avail in MP4Decoder
|
Member Function Documentation
|
Returns the buffer with U component of *YUVI420* of Uncompressed Video.
|
|
Returns the buffer with V component of *YUVI420* of Uncompressed Video.
|
|
Returns the buffer with Y component of *YUVI420* of Uncompressed Video.
|
|
References ColorSpace. Referenced by FrameRotate::adapt(), X11Renderer::open(), and SDLRenderer::open().
|
The documentation for this class was generated from the following files: