QFrameBuffer Class Reference
This class serves as a frame buffer and event handler of a QLabel widget for displaying video frames.It manages a QImage object as a frame buffer whose pixels may be set using the setPixel() method. For painting the frame buffer's contents into the QLabel widget's pixmap, a customEvent() handler is implemented.
Although this class is an extension of QWidget, it is not intended to be used as a replacement of the QLabel instance actually displaying the video frames. Actually, a QFrameBuffer widget will never become visible. . More...
#include <QFrameBuffer.hpp>
Inheritance diagram for QFrameBuffer:
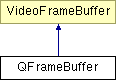
Public Member Functions | |
QFrameBuffer (QLabel *qlabel, int depth, int numColors=0, bool gray=true, QEvent::Type eventType=QEvent::User) | |
Constructor. | |
virtual | ~QFrameBuffer () |
Destructor. | |
void | setPixel (int x, int y, uint index_or_rgb) |
Set a pixel in the frame buffer. | |
void | postPaintEvent () |
Post a custom event for this object to display the frame buffer on the QLabel widget. | |
Protected Member Functions | |
virtual void | customEvent (QCustomEvent *event) |
Event handler for displaying the QImage buffer's contents in the associated QLabel widget. |
Detailed Description
This class serves as a frame buffer and event handler of a QLabel widget for displaying video frames.It manages a QImage object as a frame buffer whose pixels may be set using the setPixel() method. For painting the frame buffer's contents into the QLabel widget's pixmap, a customEvent() handler is implemented.
Although this class is an extension of QWidget, it is not intended to be used as a replacement of the QLabel instance actually displaying the video frames. Actually, a QFrameBuffer widget will never become visible. .
- Author:
- Mario Taschwer
- Version:
- Id
- QFrameBuffer.hpp,v 1.5 2005/02/22 18:26:11 mkropfbe Exp
Definition at line 75 of file QFrameBuffer.hpp.
Constructor & Destructor Documentation
|
Constructor.
|
Member Function Documentation
|
Post a custom event for This is thread-safe and will cause the GUI thread to invoke customEvent(). We need to use custom events, as hidden widgets will not receive paint events. Implements VideoFrameBuffer. Definition at line 87 of file QFrameBuffer.cpp.
|
|
Set a pixel in the frame buffer. If this method is called from a thread other than the Qt GUI thread, the frame buffer should be locked before by calling the lock() method.
Reimplemented from VideoFrameBuffer. Definition at line 108 of file QFrameBuffer.hpp.
|
The documentation for this class was generated from the following files: