SDLaudioIO Class Reference
provides raw-audio output via the SDL library methods <short description=""> More...
#include <SDLaudioIO.hpp>
Inheritance diagram for SDLaudioIO:
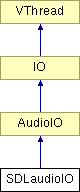
Public Member Functions | |
SDLaudioIO (AudioESInfo *es, ESSynchronizer *ess=NULL) | |
Constructor. | |
int | initialize () |
Frame * | getFrame () |
returns a frame if one complete frame is available, otherwise null is returned. | |
int | writeFrame (Frame *frm, ESInfo *out_es=NULL) |
returns the number of packets sent. | |
bool | open () |
opens the IO connection. | |
bool | close (bool immediate=false) |
closes the IO class. | |
IO::State | play (double prefetchTime=0.0) |
IO::State | pause () |
IO::State | mute () |
int | packet_queue_get (PacketQueue *q, APacket *pkt, int block) |
void | resume () |
void | clearBuffer () |
clear packet buffer | |
int | getPacketsInQueue () |
Friends | |
void | sdl_audio_callback (void *opaque, Uint8 *stream, int length) |
Detailed Description
provides raw-audio output via the SDL library methods <short description="">
- Author:
- Mithlesh Kumar
- Version:
- Id
- SDLaudioIO.hpp,v 1.13 2006/01/20 15:37:18 mkropfbe Exp
Definition at line 132 of file SDLaudioIO.hpp.
Constructor & Destructor Documentation
|
Constructor.
|
Member Function Documentation
|
closes the IO class.
Implements IO. Definition at line 587 of file SDLaudioIO.cpp. References IO::setState().
|
|
returns a frame if one complete frame is available, otherwise null is returned. This function is typically blocking. Don't use a NULL return value to conclude STREAMEOF, always check with getState()! Implements IO. Definition at line 437 of file SDLaudioIO.cpp.
|
|
opens the IO connection. State is set to OPENING. When the connection is ready for use, State is OPEN Implements IO. Definition at line 542 of file SDLaudioIO.cpp. References ESSynchronizer::setPaused(), and IO::setState().
|
|
returns the number of packets sent. Returns 0 on error Implements IO. Definition at line 450 of file SDLaudioIO.cpp. References Frame::getAU().
|
The documentation for this class was generated from the following files: