DSPaudioIO Class Reference
audio output to linux pseudo-file /dev/dsp <short description=""> More...
#include <DSPaudioIO.hpp>
Inheritance diagram for DSPaudioIO:
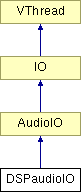
Public Member Functions | |
DSPaudioIO (AudioESInfo *es, ESSynchronizer *ess=NULL, bool enableHeadphones=false) | |
int | initialize () |
Frame * | getFrame () |
returns a frame if one complete frame is available, otherwise null is returned. | |
int | writeFrame (Frame *frm, ESInfo *out_es=NULL) |
returns the number of packets sent. | |
bool | open () |
opens the IO connection. | |
bool | close (bool immediate=false) |
closes the IO class. |
Detailed Description
audio output to linux pseudo-file /dev/dsp <short description="">
- Author:
- Mithlesh Kumar
- Version:
- Id
- DSPaudioIO.hpp,v 1.10 2006/01/20 15:37:18 mkropfbe Exp
Definition at line 78 of file DSPaudioIO.hpp.
Member Function Documentation
|
closes the IO class.
Implements IO. Definition at line 167 of file DSPaudioIO.cpp. References AudioIO::destroy(), and IO::setState().
|
|
returns a frame if one complete frame is available, otherwise null is returned. This function is typically blocking. Don't use a NULL return value to conclude STREAMEOF, always check with getState()! Implements IO. Definition at line 117 of file DSPaudioIO.cpp.
|
|
opens the IO connection. State is set to OPENING. When the connection is ready for use, State is OPEN Implements IO. Definition at line 156 of file DSPaudioIO.cpp. References ESSynchronizer::setPaused(), and IO::setState().
|
|
returns the number of packets sent. Returns 0 on error Implements IO. Definition at line 122 of file DSPaudioIO.cpp. References GlobalTimer::adjustToTS(), Frame::getAU(), ESSynchronizer::getGlobalTimer(), and ESSynchronizer::setPaused().
|
The documentation for this class was generated from the following files: