VITMuxDemuxIO Class Reference
muxes/demuxes from/to our VIT container to elementary-stream BufferIOs <short description=""> More...
#include <VITMuxDemuxIO.hpp>
Inheritance diagram for VITMuxDemuxIO:
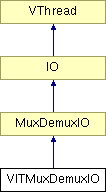
Public Member Functions | |
VITMuxDemuxIO (ByteStream *stream) | |
virtual Frame * | getFrame () |
returns a frame and forwards it to the correct BufferIO This function is typically blocking. | |
virtual int | writeFrame (Frame *frm, ESInfo *out_es=NULL) |
muxes a frame to the container |
Detailed Description
muxes/demuxes from/to our VIT container to elementary-stream BufferIOs <short description="">
- Author:
- Michael Kropfberger
- Version:
- Id
- VITMuxDemuxIO.hpp,v 1.3 2006/02/24 08:17:32 mkropfbe Exp
Definition at line 64 of file VITMuxDemuxIO.hpp.
Member Function Documentation
|
returns a frame and forwards it to the correct BufferIO This function is typically blocking. Don't use a NULL return valuse to conclude STREAMEOF, always check with getState()! Reimplemented from MuxDemuxIO. Definition at line 155 of file VITMuxDemuxIO.cpp. References MuxDemuxIO::open(), ByteStream::read(), Frame::setAU(), Frame::setStreamID(), and ByteStream::tell().
|
|
muxes a frame to the container Returns -1 on error Reimplemented from MuxDemuxIO. Definition at line 58 of file VITMuxDemuxIO.cpp. References Frame::getAU(), ESInfo::getAvgBandwidth(), ESInfo::getCodecID(), ESInfo::getStreamId(), ESInfo::getVOPTimeIncrement(), ESInfo::isAudioStream(), ESInfo::isVisualStream(), MuxDemuxIO::open(), and ByteStream::write().
|
The documentation for this class was generated from the following files: