SDLFrameBuffer Class Reference
This class serves as a frame buffer and event handler of a SDL RGB Surface widget for displaying video frames. More...
#include <SDLFrameBuffer.hpp>
Inheritance diagram for SDLFrameBuffer:
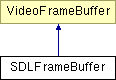
Public Member Functions | |
SDLFrameBuffer (SDL_Surface *surface, int depth, bool gray, int width, int height) | |
Constructor. | |
virtual | ~SDLFrameBuffer () |
Destructor. | |
void | setPixel (int x, int y, uint value) |
Set a pixel in the frame buffer. | |
void | bitBlt (AU *au) |
does the fast bitblitting of the full (and unscaled) Frame | |
void | lock () |
Lock the mutex protecting the frame buffer. | |
void | unlock () |
Unlock the mutex locked by lock(). | |
void | postPaintEvent () |
Post a custom event for this object to display the frame buffer on the SDL surface. | |
Protected Attributes | |
SDL_Surface * | image |
SDL_Surface * | surface |
int | width |
int | height |
Detailed Description
This class serves as a frame buffer and event handler of a SDL RGB Surface widget for displaying video frames.
- Author:
- Michael Kropfberger
- Version:
- Id
- SDLFrameBuffer.hpp,v 1.6 2005/01/16 16:35:12 mkropfbe Exp
Definition at line 61 of file SDLFrameBuffer.hpp.
Constructor & Destructor Documentation
|
Constructor.
|
Member Function Documentation
|
Lock the mutex protecting the frame buffer. This function will block the calling thread until the mutex can be obtained.
Reimplemented from VideoFrameBuffer. Definition at line 91 of file SDLFrameBuffer.hpp.
|
|
Post a custom event for This is thread-safe and will cause the GUI thread to invoke customEvent(). We need to use custom events, as hidden widgets will not receive paint events. Implements VideoFrameBuffer. Definition at line 156 of file SDLFrameBuffer.cpp.
|
|
Set a pixel in the frame buffer. the frame buffer should be locked before setPixel() by calling the lock() method. Reimplemented from VideoFrameBuffer. Definition at line 111 of file SDLFrameBuffer.cpp.
|
The documentation for this class was generated from the following files: