CacheManagerLRU Class Reference
<class description="" goes="" here=""> <short description=""> More...
#include <CacheManagerLRU.hpp>
Inheritance diagram for CacheManagerLRU:
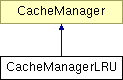
Public Member Functions | |
CacheManagerLRU (u32 size, bool serverMode) | |
void | putVideo (ContainerInfo *video, u32 dskSpaceAlreadyReserved=0) |
inserts the video, if the video is smaller than the total cache size. | |
ContainerInfo * | getVideo (const Url *URL) |
returns an ContainerInfo for the given url, if the URL is cached. | |
u32 | getCacheSize () const |
u32 | getActSize () const |
u32 | getRequests () const |
u32 | getHits () const |
u64 | getByteRequested () const |
u64 | getByteHits () const |
double | getQualityHits () const |
void | getContent () |
void | saveCacheIndexToDir (const char *dir) |
Protected Member Functions | |
bool | replace (bool testPriorDelete) |
void | internalConsistencyCheck () |
MetaObject * | findMetaObject (const Url *url) |
void | deleteMetaObject (const Url *url) |
u32 | getSizeOfNotLockedObjects () |
requires mutex to be locked | |
Protected Attributes | |
list< ContainerInfo * > | lrulist |
list of video objects, which will be replaced | |
list< MetaObject * > | metaObjects |
stores metaobjects |
Detailed Description
<class description="" goes="" here=""> <short description="">
- Author:
- Michael Kropfberger and Peter Schojer
- Version:
- Id
- CacheManagerLRU.hpp,v 1.6 2006/01/20 15:37:17 mkropfbe Exp
Definition at line 64 of file CacheManagerLRU.hpp.
Member Function Documentation
|
returns an ContainerInfo for the given url, if the URL is cached. lock count is increased by one. If the client/session object is finished with the ContainerInfo object it has to call info->unlock()!!!! Implements CacheManager. Definition at line 171 of file CacheManagerLRU.cpp. References ContainerInfo::getFirstVisualES(), ContainerInfo::getUrl(), lrulist, and Url::toString().
|
|
inserts the video, if the video is smaller than the total cache size. If it is bigger, the video will be destroyed and its memory freed! LRU doesn't take advantage of adaptation. We just have full or zero quality Implements CacheManager. Definition at line 97 of file CacheManagerLRU.cpp. References MetaObject::addMP4Stream(), MetaObject::deleteMP4Stream(), ContainerInfo::destroy(), ContainerInfo::getESList(), ContainerInfo::getFileSize(), ContainerInfo::getFirstAudioES(), ContainerInfo::getFirstVisualES(), ContainerInfo::getUrl(), ReferenceCounter::getUsage(), ContainerInfo::getUsageCounter(), lrulist, metaObjects, replace(), and Url::toString().
|
|
Implements CacheManager. Definition at line 320 of file CacheManagerLRU.cpp. References MetaObject::deleteMP4Stream(), MetaObject::getNumberOfVariations(), getSizeOfNotLockedObjects(), MetaObject::getUrl(), and lrulist. Referenced by putVideo().
|
The documentation for this class was generated from the following files: