BufferedHttpMPGStreamReader Class Reference
#include <BufferedHttpMPGStreamReader.hpp>
Inheritance diagram for BufferedHttpMPGStreamReader:
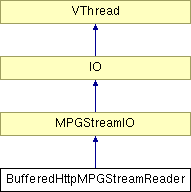
Public Member Functions | |
BufferedHttpMPGStreamReader (ESInfo *es, const char *file, bool writeOnly, bool omitHeader=false, int bufferSize=STREAMREADER_DEF_BUFFER_SIZE, int maxFrameSize=MAX_FRAME_SIZE) | |
bool | testFileopen () |
Frame * | getFrame () |
returns a frame if one complete frame is available, otherwise null is returned. | |
int | writeFrame (const Frame *frm) |
bool | open () |
opens the IO connection. | |
bool | close (bool immediate=false) |
closes the IO class. | |
bool | destroy () |
deletes the elementary stream and its extra hint-file | |
bool | setToFrameNumber (u32 frameNumber) |
repositions the IO class to the given frame. | |
long | setToClosestIFrame (u32 frameNumber, bool backwards) |
repositions to previous I-VOP, | |
u32 | getContentLength () |
Static Public Member Functions | |
int | getDefaultBufferSize () |
Detailed Description
- Author:
- Klaus Schoeffmann
Definition at line 73 of file BufferedHttpMPGStreamReader.hpp.
Member Function Documentation
|
closes the IO class.
Reimplemented from MPGStreamIO. Definition at line 112 of file BufferedHttpMPGStreamReader.cpp. References HttpRequest::closeSock(), and IO::setState(). Referenced by getFrame().
|
|
returns a frame if one complete frame is available, otherwise null is returned. This function is typically blocking. Don't use a NULL return value to conclude STREAMEOF, always check with getState()! Reimplemented from MPGStreamIO. Definition at line 230 of file BufferedHttpMPGStreamReader.cpp. References close(), ESInfo::getVOPTimeIncrement(), ESInfo::isAudioStream(), ESInfo::isVisualStream(), Frame::setAU(), and Frame::setMediaTimeScale().
|
|
opens the IO connection. State is set to OPENING. Depending on the underlying QIODevice, a network connection is stablished or a file connection. When the connection is ready for use, State is OPEN Reimplemented from MPGStreamIO. Definition at line 92 of file BufferedHttpMPGStreamReader.cpp. References HttpRequest::getContentLength(), HttpRequest::makeHttpRequest(), and IO::setState().
|
|
repositions to previous I-VOP,
Reimplemented from MPGStreamIO. Definition at line 97 of file BufferedHttpMPGStreamReader.hpp.
|
|
repositions the IO class to the given frame. Will return false in the following cases:
Reimplemented from MPGStreamIO. Definition at line 95 of file BufferedHttpMPGStreamReader.hpp.
|
The documentation for this class was generated from the following files: